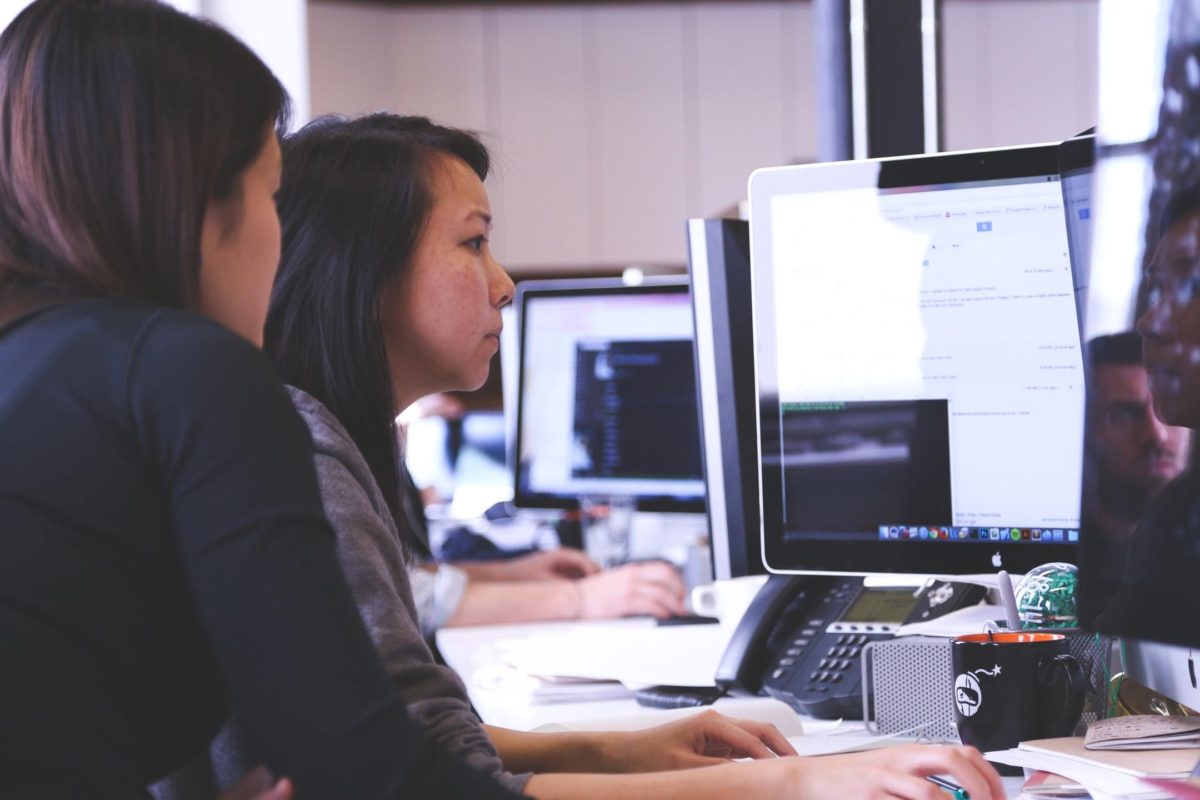
The React.js Component Lifecycle
I’ve been working in React for awhile now and I wrote a little app to solidify the component lifecycle in my own mind. None of this will be a surprise if you’ve spent much time in React yourself.
First, I created a simple component and implemented all the lifecycle components and just had them print their names to the console, which I can monitor in the dev tools of my browser.
constructor(props) { super(props); console.log('constructor'); } componentWillMount = () => { console.log('componentWillMount'); }; componentDidMount = () => { console.log('componentDidMount'); }; componentWillReceiveProps = () => { console.log('componentWillReceiveProps'); }; componentWillUpdate = () => { console.log('componentWillUpdate'); }; componentDidUpdate = () => { console.log('componentDidUpdate'); }; componentWillUnmount = () => { console.log('componentWillUnmount'); }; componentDidCatch = (error, errorInfo) => { console.log('componentDidCatch'); }; // shouldComponentUpdate = () => { console.log('shouldComponentUpdate'); return false; };
componentDidCatch is an interesting one as we should not likely be calling that directly. I just put it in to see it fire, as you can if you pull the code and uncomment that line.
First, here’s my little app.
As you can see, all we’re going to do is toggle some text or mount and unmount the component. The text is toggled by passing in a property from a parent component. When the ‘text’ property changes, the text re-renders.
So, what do I see?
When the component initially loads, I get this in my browser’s console:
When I toggle the text, passing a new property and forcing a re-render, I get this:
Why didn’t componentWillRecieveProps last time? Because the props should be managed in the constructor if needed, and this is an update to a mounted component. No mounting occurred here, only updating.
Now, let’s unmount the component:
Pretty darned simple this time, eh? Only one lifecycle method gets called, letting us now the component will soon unmount and gives us a chance to do any cleanup (but your components are stateless and you don’t need to do cleanup, right?).
Note, I commented out?shouldComponentUpdate?and it doesn’t appear in the firing order. It does indeed fire when updating a component, but in general I stay away from it as we must return a true or false from this function to indicate if the update cycle should complete. This means you would need some reason to overload the default behavior or re-rendering on state or prop change, or monitor the state of these things yourself. Yucky. I just avoid it if at all possible.
One thought on “The React.js Component Lifecycle”
Comments are closed.