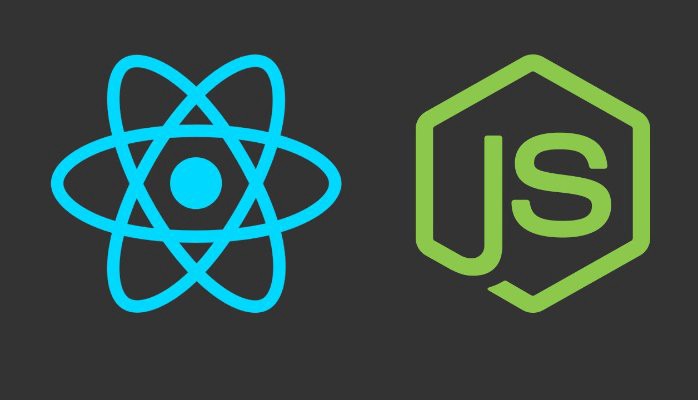
CORS your dev environment for Node.js and React.js
Building a Node.js and React.js full stack app can have its tooling annoyances, one of which is getting the Node.js server to allow your requests in from your webpack-dev-server hosted React app, which is going to be running on a different port than your Node application. There are many ways to develop around this, but this article covers what I find effective when my development environment starts out looking like the image below.
Why this happens
The Node server is doing its job well. It is preventing a call to an API endpoint from a different machine, about which it knows nothing. The Node server is guarding against a cross-site scripting attack.
Good job! But, a PITA while developing.
A static solution
Some developers just configure their client application to webpack –watch and build the client code into the Node server’s public directory. This means anytime the React.js code changes, it gets built into the Node.js server path where it will be served for production.
While this technique works, it means manually refreshing the page being served up by Node.js when my React code updates. What a pain, right? Who can stand this manual refresh business? Obviously we can’t live with this solution : )
A more reasonable solution
Middleware can be plugged into the express app in the Node server to allow these cross-site requests. Done manually, this typically looks like the following.
var allowCrossDomain = function(req, res, next) { res.header("Access-Control-Allow-Origin", "*"); // allow requests from any other server res.header('Access-Control-Allow-Methods', 'GET,PUT,POST,DELETE'); // allow these verbs res.header("Access-Control-Allow-Headers", "Origin, X-Requested-With, Content-Type, Accept, Cache-Control"); app.use(allowCrossDomain); // plumbing it in as middleware
This code could get some sort of config flag around it for production, or better still, get removed for production. In production, after all, we’ll be serving up the React app from the Node server and client requests can be made to endpoints without a servername: /api/foo_endpoint.
CORS
I found an npm package called “cors” designed to simplify and even wrap up this problem. It does far more than solve for my development scenario, and the GitHub site for cors shows its many other uses. It is highly configurable an is useful for guarding even single endpoints. The additional functionality is well documented in the repo. That said, the default options solve my little problem quite handily and save me the tedious task of looking up all that header syntax when I spool up a new Node app.
Thanks to the contributors of this very useful middleware.
Now, it’s time for me to get back to coding.
One thought on “CORS your dev environment for Node.js and React.js”
Comments are closed.