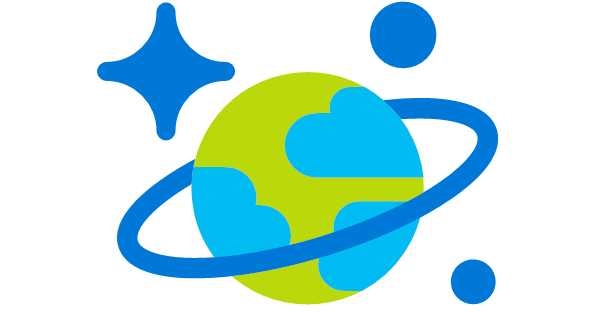
Exploring the new Cosmos DB async JavaScript API
When learning a new database API, I typically create a small script to exercises the simple CRUD operations to explore how the API is formed, its logical usage, etc. Sometimes I’ll add a few more operations exercising more complex operations. The new JavaScript SDK for Cosmo DB has been up on GitHub about 4 months now, but it took me a while to get to try it. Here I’ll share what I learned.
Firstly, the new SDK eschews callbacks in favor of async/await. Asynchronous code is a new paradigm for many JavaScript developers. Callbacks are still supported, of course, but the async/await should keep us out of callback hell.
One tidy structure we can use instead of a long callback tree is to use:
.then()
It’s basically a wrapper for us, but they line up a bit tidier for us as we exercise our Cosmos DB.
I apologize for the terrible code formats. I am trying to find a good WordPress code formatter, so if you have any tips there it would be great to get them in comments.
Adding a set of documents looks like this in SQL syntax using a batch setup so as not to insert one JSON document at a time. This technique can only take 100 docs at a time.
function addManyDocs() { const {database} = await client.databases.createIfNotExists({id: databaseId}); const {container} = await database.containers.createIfNotExists({id: containerId}); const p = []; for (i = 0; i < 100; i++) { const doc = documentFactory(); p.push(container.items.create(doc)); } await Promise.all(p); }
That was pretty easy, now let’s query those docs. Note this time we are walking the docs from the DB one at a time after getting a collection of them. Use your query to limit the number of documents returned from this call. You don’t want to try deleting 100,000 docs this way. You’d likely want to chunk them even it’s it is something like this:
SQL top 1000 *
Since we are only with 100 documents here, I’ll query for all of them and then delete them. Note that in this deleting
technique we are using, we do have to delete the docs one at a time. This makes sense, for synchronicity sake.
Remember, Cosmos can be in a globally distributed configuration and these writes and deletes can take a
few milliseconds to actually update across databases. So, the SDK designers had to provide techniques to
be safely asynchronous during writes and deletes.
async function deleteAllDocs() { const queryString = 'select * from items i where i.id != ""'; const {database} = await client.databases.createIfNotExists({id: databaseId}); const {container} = await database.containers.createIfNotExists({id: containerId}); const {result: docs} = await container.items.query(queryString).toArray(); console.log(`DELETING: ${docs.length} DOCS`); let cnt = 0; docs.map(async (doc) => { cnt++; container.item(doc.id).delete(doc); }); console.log(`DELETED: ${cnt} DOCS`); }
All of the code for this script is on GitHub and feel free to throw any feedback my way in the comments.
There are even more examples of data operations in the complete code file.
Learn more at the following references.
New Azure #CosmosDB JavaScript SDK 2.0 now in public preview
6 Reasons Why JavaScript’s Async/Await Blows Promises Away (Tutorial)
Recent Comments